Azure Queue Storage is shaking things up in the world of .NET messaging, offering a game-changing solution for developers. This cloud-based service from Microsoft Azure is transforming how we handle message queues, making your apps more scalable and resilient than ever before.
In this article, we’ll dive into the exciting world of Azure integration with CoreWCF and WCF Client. You’ll discover how to harness the power of Azure Queue Storage to boost your .NET applications. We’ll walk you through the ins and outs of integrating CoreWCF with Azure Queue Storage, and then show you how to do the same with WCF Client. By the end, you’ll have the know-how to take your messaging to the next level with Azure’s cutting-edge technology.
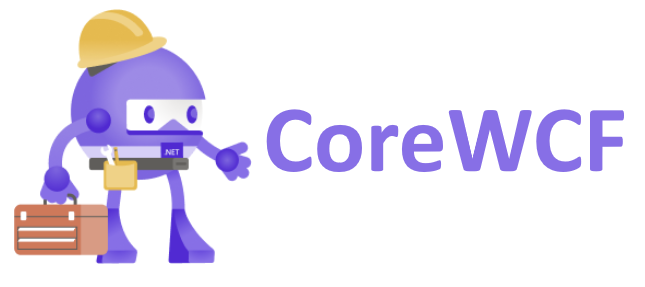
Azure Queue Storage: Revolutionizing .NET Messaging
Overview of Azure Queue Storage
Azure Queue Storage is shaking up the world of .NET messaging with its simple yet powerful approach. It’s a cloud-based service that lets you store and manage a massive number of messages, making it perfect for building flexible and scalable applications . You can access these messages from anywhere in the world using HTTP or HTTPS, giving you incredible flexibility in your app design .
One of the coolest things about Azure Queue Storage is its capacity. A single queue can hold millions of messages, and each message can be up to 64 KB in size . This means you can handle a ton of data without breaking a sweat. Plus, with the total capacity limit of a storage account, you’ve got room to grow .
Comparison with traditional messaging systems
When you stack Azure Queue Storage up against traditional messaging systems, it really shines. Here’s why:
- Scalability: Azure Queue Storage is built to handle massive workloads. It can scale to millions of messages per queue, making it perfect for high-volume applications .
- Simplicity: Unlike complex messaging systems, Azure Queue Storage offers a straightforward, lightweight service that’s easy to set up and use .
- Cost-effectiveness: If you’re looking for a budget-friendly option that doesn’t skimp on performance, Azure Queue Storage is your go-to choice .
- Durability: Your messages are in safe hands. Azure Queue Storage offers durable, reliable message storage with guaranteed delivery .
Benefits for .NET developers
As a .NET developer, you’re in for a treat with Azure Queue Storage. Here’s how it can supercharge your applications:
- Decoupling components: You can use Azure Queue Storage to separate different parts of your app, allowing them to scale independently. This makes your application more flexible and resilient .
- Asynchronous processing: Queue Storage is perfect for handling tasks in the background. You can offload time-consuming jobs from your main application, keeping it snappy and responsive .
- Burst handling: When traffic suddenly spikes, Queue Storage acts as a buffer, preventing your servers from getting overwhelmed. You can monitor queue length and add or remove worker nodes based on demand .
- Simple workflows: Building basic workflows with decoupled components becomes a breeze with Azure Queue Storage .
- Security: Microsoft invests over $1 billion annually in cybersecurity and employs more than 3,500 security experts. With Azure, you get comprehensive security and compliance built right in .
By leveraging Azure Queue Storage, you’re not just adopting a messaging system – you’re revolutionizing how your .NET applications handle communication and scaling. It’s time to take your messaging game to the next level!
CoreWCF and Azure Queue Storage Integration
Get ready to supercharge your .NET services with CoreWCF and Azure Queue Storage! This powerful combination offers a modern replacement for MSMQ, allowing your existing WCF services to communicate seamlessly with clients using Azure’s robust cloud infrastructure .
Setting up the CoreWCF Azure Queue Storage library
To kickstart your integration journey, you’ll need to install the Microsoft.CoreWCF.Azure.StorageQueues library. It’s a breeze with NuGet – just a few clicks, and you’re all set . This library is your gateway to harnessing the full potential of Azure Queue Storage in your CoreWCF services.
Configuring service endpoints
Now, let’s get your CoreWCF service talking to Azure Queue Storage. You’ll need to configure your service with the right endpoint and set up the credentials. But don’t worry, it’s easier than you might think!
Here’s how you can do it in your startup class:
public void ConfigureServices(IServiceCollection services)
public void ConfigureServices(IServiceCollection services)
{
services.AddServiceModelServices();
services.AddServiceModelConfigurationManagerFile("appsettings.json");
services.AddSingleton<IMyService, MyService>();
}
public void Configure(IApplicationBuilder app)
{
app.UseServiceModel(builder =>
{
builder.AddService<MyService>();
builder.AddServiceEndpoint<MyService, IMyService>(new AzureQueueStorageBinding(), "https://myaccount.queue.core.windows.net/myqueue");
});
}
This configuration sets up your service to receive requests from Azure Queue Storage .
Implementing message processing logic
With your service configured, it’s time to implement the logic for processing messages. Here’s a simple example of how your service interface and implementation might look:
[ServiceContract]
public interface IMyService
{
[OperationContract(IsOneWay = true)]
Task ProcessVideoAsync(long videoId, string videoTitle, string blobStoragePath);
}
public class MyService : IMyService
{
public async Task ProcessVideoAsync(long videoId, string videoTitle, string blobStoragePath)
{
await VideoEngine.ProcessVideoAsync(videoId, videoTitle, blobStoragePath);
}
}
This example shows a one-way operation for processing videos, demonstrating how you can leverage Azure Queue Storage for asynchronous tasks .
By integrating CoreWCF with Azure Queue Storage, you’re opening up a world of possibilities for your .NET services. You’ll enjoy improved scalability, reliability, and the power of cloud-based messaging. So go ahead, give it a try, and watch your services soar to new heights!
WCF Client and Azure Queue Storage Integration
Ready to supercharge your WCF client with Azure Queue Storage? Let’s dive in and see how you can seamlessly integrate these powerful technologies!
Installing the WCF Azure Queue Storage client library
To get started, you’ll need to add the WCF Azure Queue Storage client library to your project. It’s a breeze with NuGet! Here’s how:
- Open your project in Visual Studio.
- Head to the Package Manager Console.
- Type in this command: Install-Package Microsoft.WCF.Azure.StorageQueues.Client -AllowPrereleaseVersions
And just like that, you’re all set to harness the power of Azure Queue Storage in your WCF client !
Creating and configuring client proxies
Now that you’ve got the library installed, it’s time to set up your client proxies. Here’s a quick rundown: Create a binding instance for Azure Queue Storage: var aqsBinding = new AzureQueueStorageBinding();
Set up your endpoint address: string queueEndpointString = "https://MYSTORAGEACCOUNT.queue.core.windows.net/QUEUENAME";
Create a ChannelFactory and open it: var factory = new ChannelFactory<IService>(aqsBinding, new EndpointAddress(queueEndpointString));
factory.Open();
Create and open a channel:
IService channel = factory.CreateChannel();
channel.Open();
Sending messages to Azure Queue Storage
Now for the fun part – sending messages! Here’s how you can send a message to your Azure Queue Storage:
Use your channel to call your service method:
await channel.ProcessVideoAsync(videoId, videoTitle, blobStoragePath);
Don’t forget to close your channel and dispose of the factory when you’re done:
channel.Close();
await (factory as IAsyncDisposable).DisposeAsync();
And there you have it! You’ve successfully integrated WCF Client with Azure Queue Storage. Now you can send messages to your heart’s content, leveraging the power and scalability of Azure’s cloud infrastructure .
Conclusion
Azure Queue Storage is causing a revolution in .NET messaging, offering developers a powerful tool to boost their applications’ scalability and resilience. By integrating CoreWCF and WCF Client with Azure Queue Storage, you’re tapping into a world of possibilities, from handling massive workloads to building flexible, decoupled systems. This integration opens doors to improved performance, cost-effectiveness, and robust security, all backed by Microsoft’s substantial investment in cybersecurity.
As you dive into this exciting world of cloud-based messaging, remember that Azure Queue Storage isn’t just a tool – it’s a game-changer for your .NET applications. Whether you’re using CoreWCF or WCF Client, the straightforward setup process and powerful features make it a breeze to get started. So why wait? It’s time to give Azure Queue Storage a shot and see how it can take your messaging to new heights. Your apps (and your users) will thank you for it!